Arduino-radarproject
In deze Arduino-zelfstudie laat ik je zien hoe je deze coole radar kunt maken met behulp van het Arduino-bord en de Processing Development Environment. Je kunt de volgende video bekijken of de schriftelijke tutorial hieronder lezen voor meer details.
Overzicht
Alles wat je nodig hebt voor dit Arduino-project is een ultrasone sensor voor het detecteren van de objecten, een kleine servomotor voor hobbyisten om de sensor te draaien en een Arduino-bord om ze te besturen. Je kunt de volgende video bekijken of de schriftelijke tutorial hieronder lezen.[/column]
Benodigde componenten voor dit Arduino-project
U kunt deze componenten op een van de onderstaande sites krijgen:
- Ultrasone sensor HC-SR04 …………
- Servomotor………………………………..
- Arduino-bord …………………………
- Broodplank en springdraden ………
Het apparaat bouwen
- Eerst heb ik een kartonnen standaard gemaakt om de ultrasone sensor op de servomotor aan te sluiten. Ik vouwde het zoals het op de onderstaande afbeelding wordt getoond, plakte het en bevestigde het aan de servomotor met een schroef zoals deze.
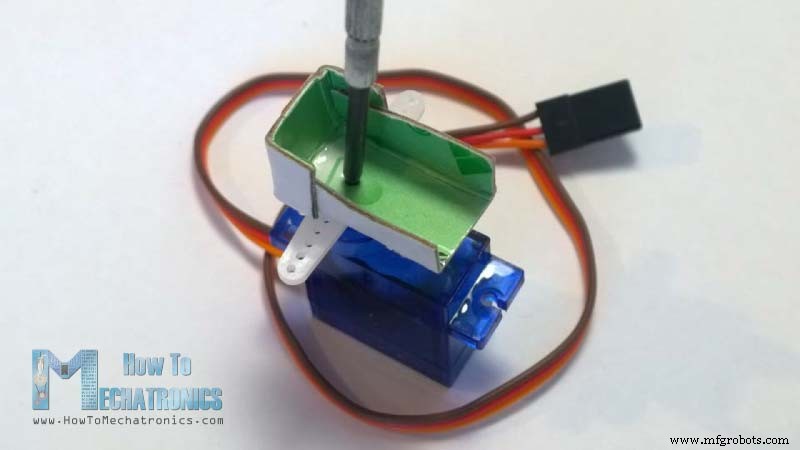
- Ik heb ook een pin-header bevestigd waarop ik 4 jumperdraden heb gesoldeerd om de sensor aan te sluiten.
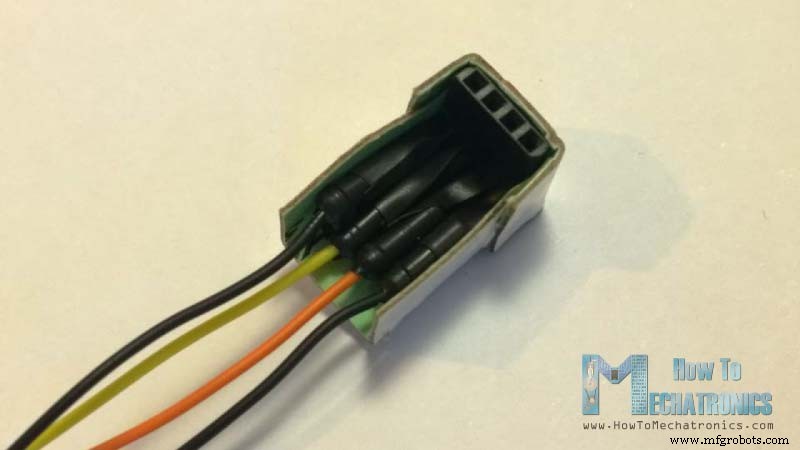
- Eindelijk heb ik de servomotor met een elastische band aan het Arduino-bord vastgemaakt.
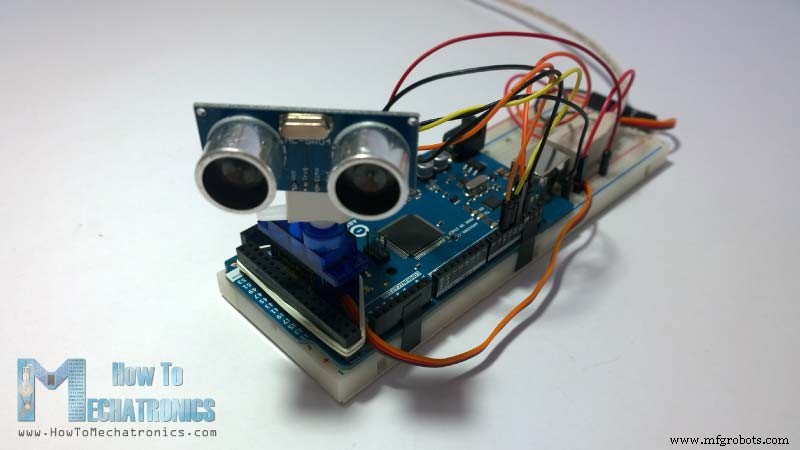
Er zijn ook een speciale montagebeugel voor de ultrasone sensor van Banggod. Je kunt ze krijgen via de volgende links:
- Ultrasone sensor met montagebeugel ………
- Montagebeugel voor ultrasoon bereik …….. Banggood
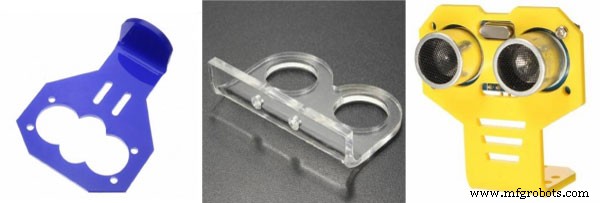
Arduino Radar Circuit Schema's
Ik heb de ultrasone sensor HC-SR04 aangesloten op pinnen 10 en 11 en de servomotor op pin 12 op het Arduino-bord.
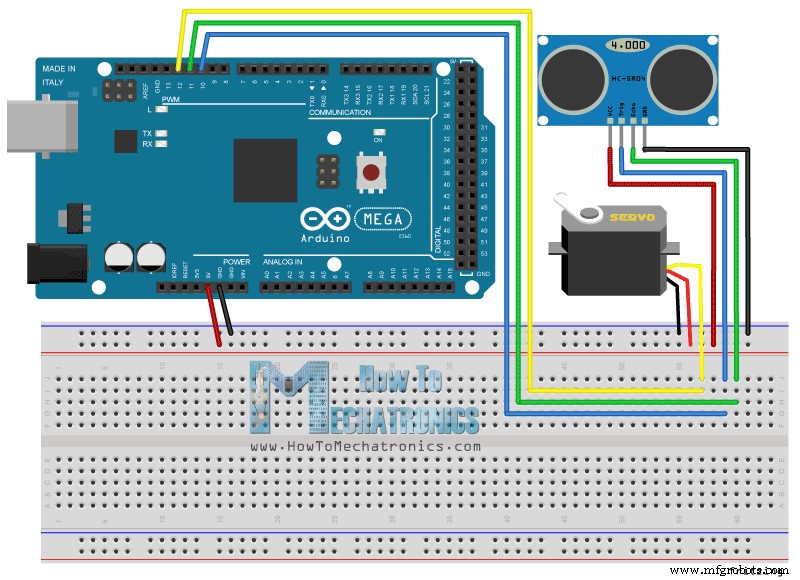
Broncodes
Nu moeten we een code maken en deze uploaden naar het Arduino-bord die de interactie tussen de Arduino en de Processing IDE mogelijk maakt. Om te begrijpen hoe de verbinding werkt, klik hier om mijn Arduino en Processing Tutorial te bezoeken.
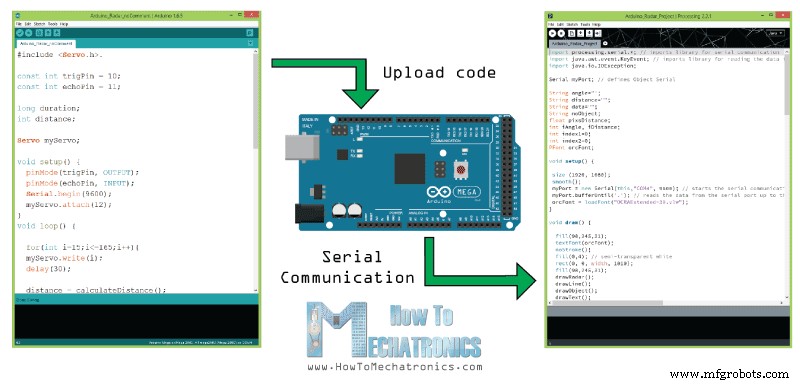
Hier is de Arduino-broncode met een beschrijving van elke regel de code:
// Includes the Servo library
#include <Servo.h>.
// Defines Tirg and Echo pins of the Ultrasonic Sensor
const int trigPin = 10;
const int echoPin = 11;
// Variables for the duration and the distance
long duration;
int distance;
Servo myServo; // Creates a servo object for controlling the servo motor
void setup() {
pinMode(trigPin, OUTPUT); // Sets the trigPin as an Output
pinMode(echoPin, INPUT); // Sets the echoPin as an Input
Serial.begin(9600);
myServo.attach(12); // Defines on which pin is the servo motor attached
}
void loop() {
// rotates the servo motor from 15 to 165 degrees
for(int i=15;i<=165;i++){
myServo.write(i);
delay(30);
distance = calculateDistance();// Calls a function for calculating the distance measured by the Ultrasonic sensor for each degree
Serial.print(i); // Sends the current degree into the Serial Port
Serial.print(","); // Sends addition character right next to the previous value needed later in the Processing IDE for indexing
Serial.print(distance); // Sends the distance value into the Serial Port
Serial.print("."); // Sends addition character right next to the previous value needed later in the Processing IDE for indexing
}
// Repeats the previous lines from 165 to 15 degrees
for(int i=165;i>15;i--){
myServo.write(i);
delay(30);
distance = calculateDistance();
Serial.print(i);
Serial.print(",");
Serial.print(distance);
Serial.print(".");
}
}
// Function for calculating the distance measured by the Ultrasonic sensor
int calculateDistance(){
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Sets the trigPin on HIGH state for 10 micro seconds
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH); // Reads the echoPin, returns the sound wave travel time in microseconds
distance= duration*0.034/2;
return distance;
}
Code language: Arduino (arduino)
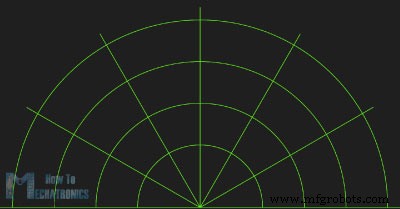
Nu ontvangen we de waarden voor de hoek en de afstand gemeten door de sensor van het Arduino-bord in de Processing IDE met behulp van de SerialEvent() functie die de gegevens van de seriële poort leest en we zullen de waarden van de hoek en de afstand in de variabelen iAngle en iDistance plaatsen. Deze variabele wordt gebruikt voor het tekenen van de radar, de lijnen, de gedetecteerde objecten en een deel van de tekst.
Voor het tekenen van de radar heb ik deze functie gemaakt drawRadar() die bestaan uit arc() en line() functies.
void drawRadar() {
pushMatrix();
translate(960,1000); // moves the starting coordinats to new location
noFill();
strokeWeight(2);
stroke(98,245,31);
// draws the arc lines
arc(0,0,1800,1800,PI,TWO_PI);
arc(0,0,1400,1400,PI,TWO_PI);
arc(0,0,1000,1000,PI,TWO_PI);
arc(0,0,600,600,PI,TWO_PI);
// draws the angle lines
line(-960,0,960,0);
line(0,0,-960*cos(radians(30)),-960*sin(radians(30)));
line(0,0,-960*cos(radians(60)),-960*sin(radians(60)));
line(0,0,-960*cos(radians(90)),-960*sin(radians(90)));
line(0,0,-960*cos(radians(120)),-960*sin(radians(120)));
line(0,0,-960*cos(radians(150)),-960*sin(radians(150)));
line(-960*cos(radians(30)),0,960,0);
popMatrix();
}
Code language: Arduino (arduino)
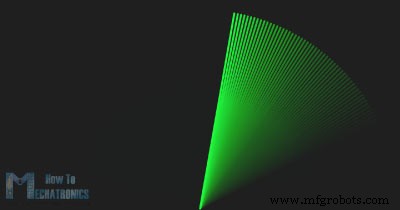
Voor het tekenen van de lijn die langs de radar beweegt, heb ik deze functie gemaakt drawLine() . Het draaipunt wordt ingesteld met de functie translate() en met de functie line() waarin de variabele iAngle wordt gebruikt, wordt de lijn voor elke graad opnieuw getekend.
void drawLine() {
pushMatrix();
strokeWeight(9);
stroke(30,250,60);
translate(960,1000); // moves the starting coordinats to new location
line(0,0,950*cos(radians(iAngle)),-950*sin(radians(iAngle))); // draws the line according to the angle
popMatrix();
}
Code language: Arduino (arduino)
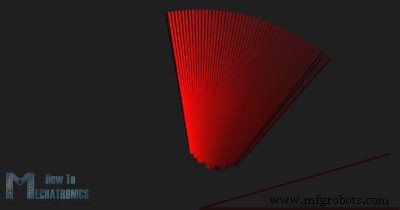
Voor het tekenen van de gedetecteerde objecten heb ik dit drawObject() . gemaakt functie. Het haalt de afstand van de ultrasone sensor, zet deze om in pixels en tekent in combinatie met de hoek van de sensor het object op de radar.
void drawObject() {
pushMatrix();
translate(960,1000); // moves the starting coordinats to new location
strokeWeight(9);
stroke(255,10,10); // red color
pixsDistance = iDistance*22.5; // covers the distance from the sensor from cm to pixels
// limiting the range to 40 cms
if(iDistance<40){
// draws the object according to the angle and the distance
line(pixsDistance*cos(radians(iAngle)),-pixsDistance*sin(radians(iAngle)),950*cos(radians(iAngle)),-950*sin(radians(iAngle)));
}
popMatrix();
}
Code language: Arduino (arduino)
Voor de tekst op het scherm heb ik de drawText() . gemaakt functie die teksten op bepaalde locaties tekent.
Al deze functies worden aangeroepen in het hoofdmenu draw() functie die zich de hele tijd herhaalt en het scherm tekent. Ook hier gebruik ik dezefill() functie met 2 parameters voor het simuleren van bewegingsonscherpte en langzame vervaging van de bewegende lijn.
void draw() {
fill(98,245,31);
textFont(orcFont);
// simulating motion blur and slow fade of the moving line
noStroke();
fill(0,4);
rect(0, 0, width, 1010);
fill(98,245,31); // green color
// calls the functions for drawing the radar
drawRadar();
drawLine();
drawObject();
drawText();
}
Code language: Arduino (arduino)
Hier is de laatste verschijning van de radar:
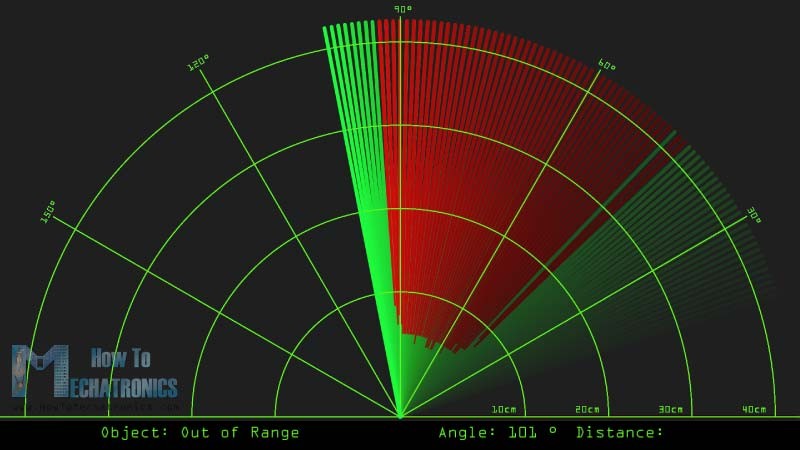
Hier is de volledige verwerkingsbroncode van de Arduino-radar:
import processing.serial.*; // imports library for serial communication
import java.awt.event.KeyEvent; // imports library for reading the data from the serial port
import java.io.IOException;
Serial myPort; // defines Object Serial
// defubes variables
String angle="";
String distance="";
String data="";
String noObject;
float pixsDistance;
int iAngle, iDistance;
int index1=0;
int index2=0;
PFont orcFont;
void setup() {
size (1920, 1080);
smooth();
myPort = new Serial(this,"COM4", 9600); // starts the serial communication
myPort.bufferUntil('.'); // reads the data from the serial port up to the character '.'. So actually it reads this: angle,distance.
orcFont = loadFont("OCRAExtended-30.vlw");
}
void draw() {
fill(98,245,31);
textFont(orcFont);
// simulating motion blur and slow fade of the moving line
noStroke();
fill(0,4);
rect(0, 0, width, 1010);
fill(98,245,31); // green color
// calls the functions for drawing the radar
drawRadar();
drawLine();
drawObject();
drawText();
}
void serialEvent (Serial myPort) { // starts reading data from the Serial Port
// reads the data from the Serial Port up to the character '.' and puts it into the String variable "data".
data = myPort.readStringUntil('.');
data = data.substring(0,data.length()-1);
index1 = data.indexOf(","); // find the character ',' and puts it into the variable "index1"
angle= data.substring(0, index1); // read the data from position "0" to position of the variable index1 or thats the value of the angle the Arduino Board sent into the Serial Port
distance= data.substring(index1+1, data.length()); // read the data from position "index1" to the end of the data pr thats the value of the distance
// converts the String variables into Integer
iAngle = int(angle);
iDistance = int(distance);
}
void drawRadar() {
pushMatrix();
translate(960,1000); // moves the starting coordinats to new location
noFill();
strokeWeight(2);
stroke(98,245,31);
// draws the arc lines
arc(0,0,1800,1800,PI,TWO_PI);
arc(0,0,1400,1400,PI,TWO_PI);
arc(0,0,1000,1000,PI,TWO_PI);
arc(0,0,600,600,PI,TWO_PI);
// draws the angle lines
line(-960,0,960,0);
line(0,0,-960*cos(radians(30)),-960*sin(radians(30)));
line(0,0,-960*cos(radians(60)),-960*sin(radians(60)));
line(0,0,-960*cos(radians(90)),-960*sin(radians(90)));
line(0,0,-960*cos(radians(120)),-960*sin(radians(120)));
line(0,0,-960*cos(radians(150)),-960*sin(radians(150)));
line(-960*cos(radians(30)),0,960,0);
popMatrix();
}
void drawObject() {
pushMatrix();
translate(960,1000); // moves the starting coordinats to new location
strokeWeight(9);
stroke(255,10,10); // red color
pixsDistance = iDistance*22.5; // covers the distance from the sensor from cm to pixels
// limiting the range to 40 cms
if(iDistance<40){
// draws the object according to the angle and the distance
line(pixsDistance*cos(radians(iAngle)),-pixsDistance*sin(radians(iAngle)),950*cos(radians(iAngle)),-950*sin(radians(iAngle)));
}
popMatrix();
}
void drawLine() {
pushMatrix();
strokeWeight(9);
stroke(30,250,60);
translate(960,1000); // moves the starting coordinats to new location
line(0,0,950*cos(radians(iAngle)),-950*sin(radians(iAngle))); // draws the line according to the angle
popMatrix();
}
void drawText() { // draws the texts on the screen
pushMatrix();
if(iDistance>40) {
noObject = "Out of Range";
}
else {
noObject = "In Range";
}
fill(0,0,0);
noStroke();
rect(0, 1010, width, 1080);
fill(98,245,31);
textSize(25);
text("10cm",1180,990);
text("20cm",1380,990);
text("30cm",1580,990);
text("40cm",1780,990);
textSize(40);
text("Object: " + noObject, 240, 1050);
text("Angle: " + iAngle +" °", 1050, 1050);
text("Distance: ", 1380, 1050);
if(iDistance<40) {
text(" " + iDistance +" cm", 1400, 1050);
}
textSize(25);
fill(98,245,60);
translate(961+960*cos(radians(30)),982-960*sin(radians(30)));
rotate(-radians(-60));
text("30°",0,0);
resetMatrix();
translate(954+960*cos(radians(60)),984-960*sin(radians(60)));
rotate(-radians(-30));
text("60°",0,0);
resetMatrix();
translate(945+960*cos(radians(90)),990-960*sin(radians(90)));
rotate(radians(0));
text("90°",0,0);
resetMatrix();
translate(935+960*cos(radians(120)),1003-960*sin(radians(120)));
rotate(radians(-30));
text("120°",0,0);
resetMatrix();
translate(940+960*cos(radians(150)),1018-960*sin(radians(150)));
rotate(radians(-60));
text("150°",0,0);
popMatrix();
}
Code language: Arduino (arduino)
Nieuwe bijgewerkte versie van de Arduino Radar-code voor elke schermresolutie:
Verander gewoon de waarden in de size() functie, met uw schermresolutie.
/* Arduino Radar Project
*
* Updated version. Fits any screen resolution!
* Just change the values in the size() function,
* with your screen resolution.
*
* by Dejan Nedelkovski,
* www.HowToMechatronics.com
*
*/
import processing.serial.*; // imports library for serial communication
import java.awt.event.KeyEvent; // imports library for reading the data from the serial port
import java.io.IOException;
Serial myPort; // defines Object Serial
// defubes variables
String angle="";
String distance="";
String data="";
String noObject;
float pixsDistance;
int iAngle, iDistance;
int index1=0;
int index2=0;
PFont orcFont;
void setup() {
size (1920, 1080); // ***CHANGE THIS TO YOUR SCREEN RESOLUTION***
smooth();
myPort = new Serial(this,"COM4", 9600); // starts the serial communication
myPort.bufferUntil('.'); // reads the data from the serial port up to the character '.'. So actually it reads this: angle,distance.
orcFont = loadFont("OCRAExtended-30.vlw");
}
void draw() {
fill(98,245,31);
textFont(orcFont);
// simulating motion blur and slow fade of the moving line
noStroke();
fill(0,4);
rect(0, 0, width, height-height*0.065);
fill(98,245,31); // green color
// calls the functions for drawing the radar
drawRadar();
drawLine();
drawObject();
drawText();
}
void serialEvent (Serial myPort) { // starts reading data from the Serial Port
// reads the data from the Serial Port up to the character '.' and puts it into the String variable "data".
data = myPort.readStringUntil('.');
data = data.substring(0,data.length()-1);
index1 = data.indexOf(","); // find the character ',' and puts it into the variable "index1"
angle= data.substring(0, index1); // read the data from position "0" to position of the variable index1 or thats the value of the angle the Arduino Board sent into the Serial Port
distance= data.substring(index1+1, data.length()); // read the data from position "index1" to the end of the data pr thats the value of the distance
// converts the String variables into Integer
iAngle = int(angle);
iDistance = int(distance);
}
void drawRadar() {
pushMatrix();
translate(width/2,height-height*0.074); // moves the starting coordinats to new location
noFill();
strokeWeight(2);
stroke(98,245,31);
// draws the arc lines
arc(0,0,(width-width*0.0625),(width-width*0.0625),PI,TWO_PI);
arc(0,0,(width-width*0.27),(width-width*0.27),PI,TWO_PI);
arc(0,0,(width-width*0.479),(width-width*0.479),PI,TWO_PI);
arc(0,0,(width-width*0.687),(width-width*0.687),PI,TWO_PI);
// draws the angle lines
line(-width/2,0,width/2,0);
line(0,0,(-width/2)*cos(radians(30)),(-width/2)*sin(radians(30)));
line(0,0,(-width/2)*cos(radians(60)),(-width/2)*sin(radians(60)));
line(0,0,(-width/2)*cos(radians(90)),(-width/2)*sin(radians(90)));
line(0,0,(-width/2)*cos(radians(120)),(-width/2)*sin(radians(120)));
line(0,0,(-width/2)*cos(radians(150)),(-width/2)*sin(radians(150)));
line((-width/2)*cos(radians(30)),0,width/2,0);
popMatrix();
}
void drawObject() {
pushMatrix();
translate(width/2,height-height*0.074); // moves the starting coordinats to new location
strokeWeight(9);
stroke(255,10,10); // red color
pixsDistance = iDistance*((height-height*0.1666)*0.025); // covers the distance from the sensor from cm to pixels
// limiting the range to 40 cms
if(iDistance<40){
// draws the object according to the angle and the distance
line(pixsDistance*cos(radians(iAngle)),-pixsDistance*sin(radians(iAngle)),(width-width*0.505)*cos(radians(iAngle)),-(width-width*0.505)*sin(radians(iAngle)));
}
popMatrix();
}
void drawLine() {
pushMatrix();
strokeWeight(9);
stroke(30,250,60);
translate(width/2,height-height*0.074); // moves the starting coordinats to new location
line(0,0,(height-height*0.12)*cos(radians(iAngle)),-(height-height*0.12)*sin(radians(iAngle))); // draws the line according to the angle
popMatrix();
}
void drawText() { // draws the texts on the screen
pushMatrix();
if(iDistance>40) {
noObject = "Out of Range";
}
else {
noObject = "In Range";
}
fill(0,0,0);
noStroke();
rect(0, height-height*0.0648, width, height);
fill(98,245,31);
textSize(25);
text("10cm",width-width*0.3854,height-height*0.0833);
text("20cm",width-width*0.281,height-height*0.0833);
text("30cm",width-width*0.177,height-height*0.0833);
text("40cm",width-width*0.0729,height-height*0.0833);
textSize(40);
text("Object: " + noObject, width-width*0.875, height-height*0.0277);
text("Angle: " + iAngle +" °", width-width*0.48, height-height*0.0277);
text("Distance: ", width-width*0.26, height-height*0.0277);
if(iDistance<40) {
text(" " + iDistance +" cm", width-width*0.225, height-height*0.0277);
}
textSize(25);
fill(98,245,60);
translate((width-width*0.4994)+width/2*cos(radians(30)),(height-height*0.0907)-width/2*sin(radians(30)));
rotate(-radians(-60));
text("30°",0,0);
resetMatrix();
translate((width-width*0.503)+width/2*cos(radians(60)),(height-height*0.0888)-width/2*sin(radians(60)));
rotate(-radians(-30));
text("60°",0,0);
resetMatrix();
translate((width-width*0.507)+width/2*cos(radians(90)),(height-height*0.0833)-width/2*sin(radians(90)));
rotate(radians(0));
text("90°",0,0);
resetMatrix();
translate(width-width*0.513+width/2*cos(radians(120)),(height-height*0.07129)-width/2*sin(radians(120)));
rotate(radians(-30));
text("120°",0,0);
resetMatrix();
translate((width-width*0.5104)+width/2*cos(radians(150)),(height-height*0.0574)-width/2*sin(radians(150)));
rotate(radians(-60));
text("150°",0,0);
popMatrix();
}
Code language: Arduino (arduino)
Productieproces
- Maak Monitor Ambilight met Arduino
- Arduino Pong Game - OLED-scherm
- Obstakels vermijden robot met servomotor
- Audiofrequentiedetector
- Arduino Tamagotchi Clone - Digital Pet
- Draadloze lichtschakelaarmanipulator
- Tech-TicTacToe
- Bewegingssensor-waterpistool
- Arduino Countdown Timer
- Arduino-zonnebloem:een elektronische zonnedanser
- RC Porsche Car (Arduino Project)